Are you a fan of Angular, but find your app’s performance to be lacking? Fear not, for there are ways to optimize your Angular application and improve its responsiveness. By following some best practices and utilizing various techniques, you can significantly enhance the performance of your app. From leveraging lazy loading and minifying your code to optimizing your components and using efficient change detection, there are many ways to optimize your Angular app. So, put on your nerd glasses and let’s dive into the world of Angular performance optimization!
- Optimize Change Detection with OnPush
Angular’s change detection mechanism is a fundamental aspect of the framework’s performance. By default, Angular uses the “Default” change detection strategy, which can be expensive as it checks all components and their children for changes on every tick. A better approach is to use the “OnPush” strategy, which only checks a component’s input bindings and triggers change detection when the reference changes. This can significantly reduce the number of checks performed during a tick, leading to faster application performance.
- Minimize Bundle Size with Lazy Loading
As your Angular application grows in size and complexity, its bundle size can become a significant factor in its performance. One way to minimize the bundle size is by using lazy loading. This technique allows you to load only the necessary parts of your application when they are needed, reducing the initial load time and improving the overall performance.
- Use AOT Compilation
Ahead-of-Time (AOT) compilation is a technique that precompiles your Angular templates into efficient JavaScript code before the application is deployed. This can lead to faster initial load times and improve the application’s overall performance.
- Optimize Rendering Performance
Rendering performance is a critical aspect of Angular performance optimization. One way to optimize rendering performance is to use the ngIf directive instead of ngShow or ngHide. ngIf removes elements from the DOM when they are not needed, while ngShow and ngHide only change the element’s visibility. This can significantly reduce the number of elements in the DOM, leading to faster rendering performance.
- Implement Change Detection Strategies
Angular provides several change detection strategies that can help optimize the performance of your application. For example, you can use the ChangeDetectorRef API to manually trigger change detection only when necessary. You can also use the async pipe to automatically unsubscribe from observables when the component is destroyed, reducing memory leaks and improving performance.
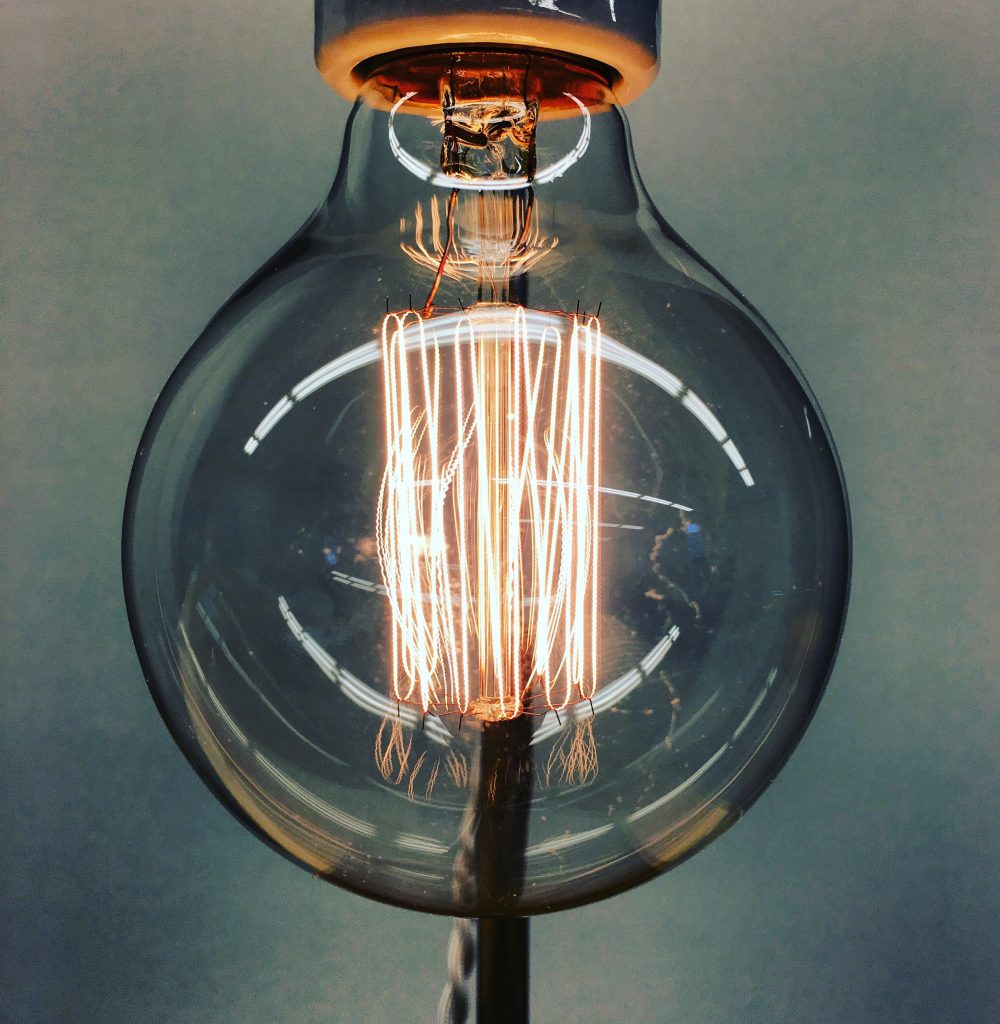
Photo by Alex Knight on Unsplash
- Optimize Network Requests
Network requests are another factor that can impact Angular performance. To optimize network requests, you can use techniques such as HTTP caching, pagination, and lazy loading of data. HTTP caching allows you to cache responses and avoid unnecessary network requests, while pagination and lazy loading allow you to load data in smaller chunks, reducing the amount of data transferred over the network.
- Use Performance Profiling Tools
Finally, you can use performance profiling tools to identify performance bottlenecks in your application. Tools such as Chrome DevTools, Angular Performance Profiler, and Augury can help you identify slow components and functions, as well as memory leaks and other issues that can impact performance.
In conclusion, optimizing Angular performance is a critical aspect of building fast and responsive web applications. By using the tips and tricks outlined above, you can significantly improve your application’s performance, providing a better user experience and making your application more competitive in today’s fast-paced digital landscape.
- Use TrackBy Function
When working with lists in Angular, you may notice that the performance can become slow when adding, removing or updating items. This is because Angular uses object identity to track changes in the list. To improve the performance, you can use the trackBy function. This function allows Angular to track items by a unique identifier, which can be faster than tracking by object identity.
- Optimize Component Initialization
Component initialization can also have an impact on Angular performance. To optimize component initialization, you can use the ngOnInit lifecycle hook instead of the constructor. The ngOnInit hook is called after the constructor and allows you to perform initialization tasks after the component is fully initialized.
- Use ChangeDetectionStrategy.OnPush and Immutable Objects
In addition to using the OnPush change detection strategy, you can also improve performance by using immutable objects. Immutable objects are objects whose state cannot be changed after they are created. When using immutable objects, you can change the reference to the object instead of modifying its state. This allows Angular to detect changes more efficiently and can improve performance.
- Optimize CSS and HTML
Finally, optimizing CSS and HTML can also have a significant impact on Angular performance. To optimize CSS, you can use tools such as PostCSS or Sass to minimize the size of your CSS files. You can also use CSS media queries to load different styles for different devices and screen sizes, reducing the amount of CSS that needs to be loaded.
To optimize HTML, you can use tools such as HTML minifiers to remove unnecessary whitespace and comments from your HTML files. You can also use the Angular compiler to remove unused components, directives, and pipes from your application.
In conclusion, optimizing Angular performance requires a combination of techniques and strategies. By using the tips and tricks outlined above, you can significantly improve the performance of your Angular application, providing a faster and more responsive user experience. Remember to use performance profiling tools to identify bottlenecks and continually monitor and optimize your application’s performance over time. With these best practices in mind, you can build fast and efficient Angular applications that provide a superior user experience.