In modern web development, security is a critical concern. Web applications store sensitive data and process important transactions, and as such, they need to be protected from unauthorized access. Authentication and authorization are two essential components of web application security. Angular is a popular framework for building single-page applications, and it comes with built-in support for authentication and authorization. In this article, we will provide a complete guide to authentication and authorization in Angular.
Authentication and Authorization: What’s the Difference?
Authentication and authorization are two distinct concepts that are often used interchangeably, but they serve different purposes. Authentication is the process of verifying the identity of a user or application. In web development, authentication typically involves asking users for a username and password, and then comparing these credentials with a database of authorized users. Once the user’s identity is confirmed, the application grants access to protected resources.
Authorization, on the other hand, is the process of determining whether a user or application is allowed to access a particular resource. Authorization is based on the user’s role or permissions. For example, a user who is authenticated as an administrator may have access to more resources than a regular user.
Authentication in Angular
Angular provides several options for implementing authentication in your application. The most common method is token-based authentication using JSON Web Tokens (JWTs). JWTs are a standard format for securely transmitting information between parties. They consist of three parts: a header, a payload, and a signature. The payload contains the user’s identity and any additional information that the application needs to verify the user’s authorization.
To implement token-based authentication in Angular, you need to perform the following steps:
Step 1: Create a Login Component
The first step is to create a login component that allows users to enter their credentials. The login component should contain a form that collects the user’s username and password. Once the user submits the form, the login component should send an HTTP request to the server to authenticate the user’s credentials.
Step 2: Verify the User’s Credentials
On the server side, you need to verify the user’s credentials against a database of authorized users. If the user’s credentials are valid, the server should generate a JWT and return it to the client. The JWT should contain the user’s identity and any additional information that the application needs to verify the user’s authorization.
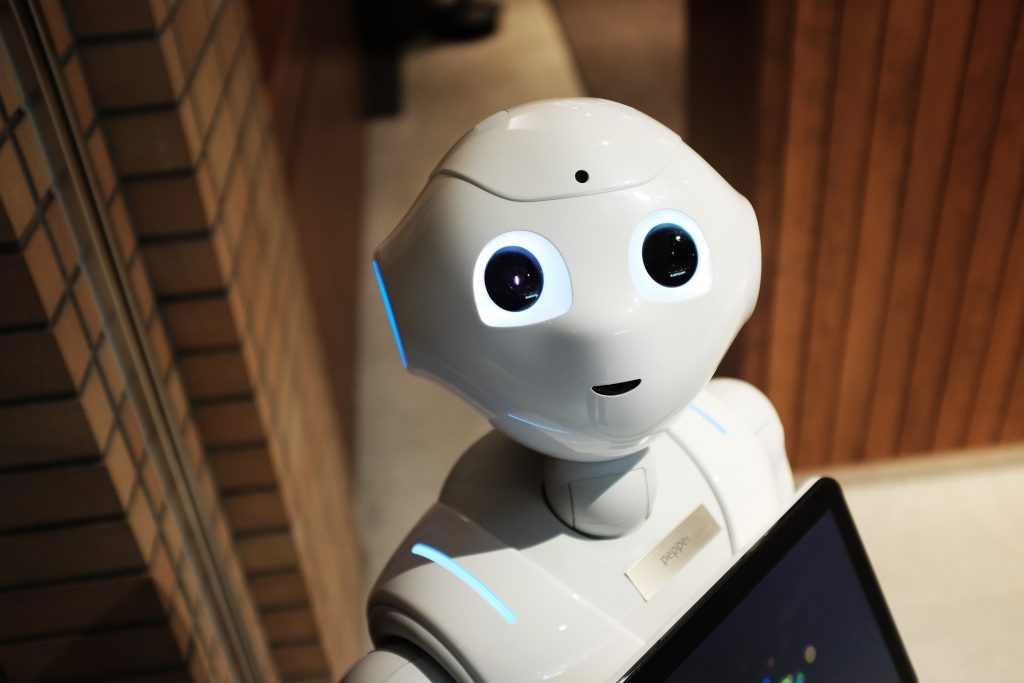
Photo by Alex Knight on Unsplash
Step 3: Store the JWT
Once the client receives the JWT, it should store it in local storage or a cookie. The JWT should be sent with every subsequent request to the server to authenticate the user’s identity.
Step 4: Create an Authentication Service
To manage authentication in your Angular application, you need to create an authentication service that handles the login and logout functionality. The authentication service should contain methods for logging in, logging out, and checking the user’s authentication status.
Step 5: Protect Your Routes
To protect your routes, you need to create a guard that checks whether the user is authenticated before allowing access to the protected route. Angular provides a built-in canActivate guard that can be used for this purpose.
Authorization in Angular
Once a user is authenticated, the application needs to determine whether the user is authorized to access a particular resource. Authorization in Angular is typically based on the user’s role or permissions. Angular provides several options for implementing authorization in your application.
Role-based Authorization
In role-based authorization, access to resources is based on the user’s role. For example, an administrator may have access to more resources than a regular user. To implement role-based authorization in Angular, you need to perform the following steps:
Step 1: Define User Roles
The first step is to define user roles in your application. This can be done by creating an enumeration that defines the different roles that are available.
Step 2: Assign Roles to Users
Next,
you need to assign roles to users. This can be done on the server side when the user logs in. The server should return the user’s role along with the JWT.
Step 3: Create an Authorization Service
To manage authorization in your Angular application, you need to create an authorization service that handles the role-based authorization logic. The authorization service should contain methods for checking whether the user is authorized to access a particular resource based on their role.
Step 4: Protect Your Routes
To protect your routes based on the user’s role, you need to create a guard that checks whether the user is authorized to access the protected route. The guard should use the authorization service to check the user’s role before allowing access to the protected route.
Permission-based Authorization
In permission-based authorization, access to resources is based on the user’s permissions. For example, a user may have permission to create, read, update, or delete resources. To implement permission-based authorization in Angular, you need to perform the following steps:
Step 1: Define User Permissions
The first step is to define user permissions in your application. This can be done by creating an enumeration that defines the different permissions that are available.
Step 2: Assign Permissions to Users
Next, you need to assign permissions to users. This can be done on the server side when the user logs in. The server should return the user’s permissions along with the JWT.
Step 3: Create an Authorization Service
To manage authorization in your Angular application, you need to create an authorization service that handles the permission-based authorization logic. The authorization service should contain methods for checking whether the user has a particular permission.
Step 4: Protect Your Routes
To protect your routes based on the user’s permissions, you need to create a guard that checks whether the user has the necessary permissions to access the protected route. The guard should use the authorization service to check the user’s permissions before allowing access to the protected route.
Conclusion
In this article, we have provided a complete guide to authentication and authorization in Angular. We have discussed the difference between authentication and authorization, and we have outlined the steps for implementing token-based authentication and role-based and permission-based authorization in Angular. By following the steps outlined in this article, you can ensure that your Angular application is secure and protected from unauthorized access.